Note
Go to the end to download the full example code.
A first 1D spring system
o---/\/\/\---o---/\/\/\---o
x---/\/\/\---o o--->
o-------/\/\/\/\/\--------o
x ... fixed node
o ... free node (moving in x-direction)
Initialization
from femedu.domain import *
from femedu.elements.linear import Spring
Building the model
Initializing a model
model = System()
Defining nodes
nd1 = Node(0.0, 0.0)
nd2 = Node(2.0, 0.0)
nd3 = Node(4.0, 0.0)
nd4 = Node(6.0, 0.0)
model.addNode(nd1, nd2, nd3, nd4)
Creating the springs
springA = Spring(nd1, nd2, 15)
springB = Spring(nd2, nd3, 10)
springC = Spring(nd3, nd4, 10)
springD = Spring(nd2, nd4, 10)
model.addElement(springA,springB,springC,springD)
Applying the essential boundary conditions
nd1.fixDOF('ux')
Applying loads
nd4.setLoad([2.0],['ux'])
You may check your model any time by executing
model.report()
System Analysis Report
=======================
Nodes:
---------------------
Node_0:
x: [0.000 0.000]
fix: ['ux']
u: None
Node_1:
x: [2.000 0.000]
u: None
Node_2:
x: [4.000 0.000]
u: None
Node_3:
x: [6.000 0.000]
P: [2.000]
u: None
Elements:
---------------------
Spring Elem_0: Node_0 to Node_1 with c=15
length change: delta = 0.0
internal force: force = 0.0
Spring Elem_1: Node_1 to Node_2 with c=10
length change: delta = 0.0
internal force: force = 0.0
Spring Elem_2: Node_2 to Node_3 with c=10
length change: delta = 0.0
internal force: force = 0.0
Spring Elem_3: Node_1 to Node_3 with c=10
length change: delta = 0.0
internal force: force = 0.0
Performing the analysis
Assembly and solve
model.solve()
Check out displacements and internal forces
model.report()
System Analysis Report
=======================
Nodes:
---------------------
Node_0:
x: [0.000 0.000]
fix: ['ux']
u: [0.000]
Node_1:
x: [2.000 0.000]
u: [0.133]
Node_2:
x: [4.000 0.000]
u: [0.200]
Node_3:
x: [6.000 0.000]
P: [2.000]
u: [0.267]
Elements:
---------------------
Spring Elem_0: Node_0 to Node_1 with c=15
length change: delta = 0.13333333333333333
internal force: force = 2.0
Spring Elem_1: Node_1 to Node_2 with c=10
length change: delta = 0.06666666666666668
internal force: force = 0.6666666666666667
Spring Elem_2: Node_2 to Node_3 with c=10
length change: delta = 0.06666666666666665
internal force: force = 0.6666666666666665
Spring Elem_3: Node_1 to Node_3 with c=10
length change: delta = 0.13333333333333333
internal force: force = 1.3333333333333333
We can also create a force plot, though it doesn’t look all that nice in 1D
model.beamValuePlot('f')
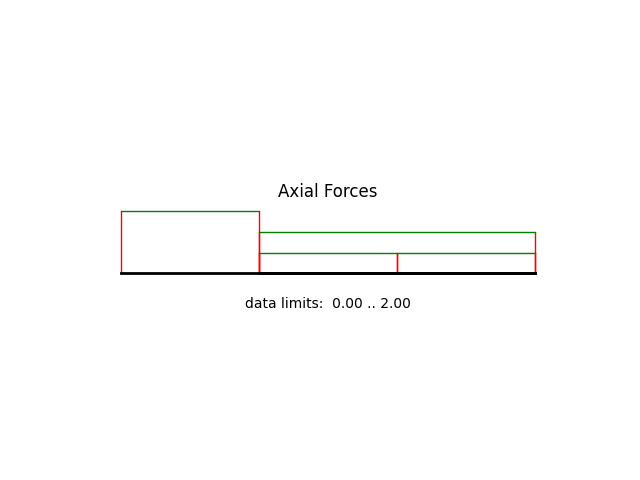
Total running time of the script: (0 minutes 0.149 seconds)