Example: plate01
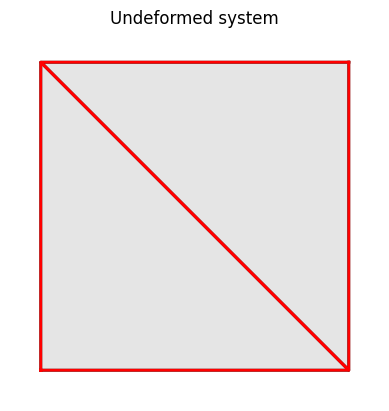
Initial system and meshing for the patch test.
We build the model based a few parameters as follows.
1 params = dict(
2 E = 10., # Young's modulus
3 nu = 0.3, # Poisson's ratio
4 t = 1.0, # thickness of the plate
5 fy = 1.e30 # yield stress
6 )
7
8 a = 10. # length of the plate in the x-direction
9 b = 10. # length of the plate in the y-direction
All mesh creation is based solely on the above parameters to allow for easy manipulation of the model.
The actual model is built by the block below.
10 model = System()
11
12 nd0 = Node( 0.0, 0.0)
13 nd1 = Node( a, 0.0)
14 nd2 = Node( a, b)
15 nd3 = Node( 0.0, b)
16
17 model.addNode(nd0, nd1, nd2, nd3)
18
19 elemA = LinearTriangle(nd0, nd1, nd3, PlaneStress(params))
20 elemB = LinearTriangle(nd2, nd3, nd1, PlaneStress(params))
21
22 model.addElement(elemA, elemB)
23
24 elemB.setSurfaceLoad(face=2, w=1.0)
25
26 model.plot(title="Undeformed system", filename="plate01_undeformed.png")
Line 9 instantiates one model space.
Lines 11-14 create the nodes, and lines 16 adds them to the model space.
Lines 18-19 create the elements and line 21 adds them to the model space. You only need to create variables for Node and Element objects, respectively, if you need to either add or retrieve information from that object later.
Line 23 adds a surface load to face 2 of element elemB
. See Triangle class
for the definition of faces for this element.
Set the load factor to 1.00 to activate and check element loads, and prescribe all degrees of freedom to eliminate the need for solving the system equations.
27 model.setLoadFactor(1.0)
28
29 nd0.setDisp( [0.0, 0.0] )
30 nd1.setDisp( [5.0, 0.0] )
31 nd2.setDisp( [5.0,-5.0] )
32 nd3.setDisp( [0.0,-5.0] )
Force the elements to update their state of strain and stress based on the prescribed nodal displacements and generate a report.
32 elemA.updateState()
33 elemB.updateState()
34
35 model.report()
Here the generated report (release: 3/12/2023):
System Analysis Report ======================= Nodes: --------------------- Node_0: x: [0. 0.] u: [0. 0.] Node_1: x: [10. 0.] u: [5. 0.] Node_2: x: [10. 10.] u: [ 5. -5.] Node_3: x: [ 0. 10.] u: [ 0. -5.] Elements: --------------------- LinearTriangle: nodes ( Node_0 Node_1 Node_3 ) material: PlaneStress strain: xx=6.250e-01 yy=-3.750e-01 xy=0.000e+00 zz=-7.500e-02 stress: xx=5.632e+00 yy=-2.060e+00 xy=0.000e+00 zz=0.000e+00 LinearTriangle: nodes ( Node_2 Node_3 Node_1 ) material: PlaneStress strain: xx=6.250e-01 yy=-3.750e-01 xy=0.000e+00 zz=-7.500e-02 stress: xx=5.632e+00 yy=-2.060e+00 xy=0.000e+00 zz=0.000e+00 element forces added to node: Node_2: [5. 0.] Node_3: [0. 0.] Node_1: [5. 0.]
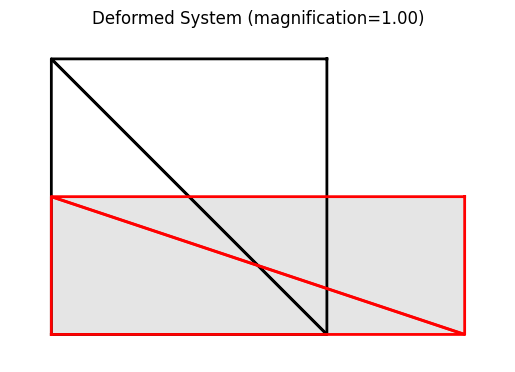
Deformed system at load level 1.00
Importing the example
from femedu.examples.plates.plate01 import *
# load the example
ex = ExamplePlate01()
More frame examples: Plate Examples