Example: truss03
We build the model based a few parameters as follows.
1 # initialize a system model
2 B = 6.0 * 12
3 H = 3.0 * 12
4 params = {'E': 10., 'A': 1., 'nu': 0.0, 'fy': 1.e30}
All mesh creation is based solely on the above parameters to allow for easy manipulation of the model.
The actual model is built by the block below.
5 model = System()
6
7 # create nodes
8 nd0 = Node(0.0, 0.0)
9 nd1 = Node( B, 0.0)
10 nd2 = Node(0.5*B, H)
11
12 model += nd0
13 model += nd1
14 model += nd2
15
16 # create elements
17 model += Truss(nd0, nd1, FiberMaterial(params)) # bottom 1
18 model += Truss(nd0, nd2, FiberMaterial(params)) # up right diag 1
19 model += Truss(nd1, nd2, FiberMaterial(params)) # up left diag 1
20
21 # define support(s)
22 nd0.fixDOF('ux') # horizontal support left end
23 #nd0 //= 0
24 nd0.fixDOF('uy') # vertical support left end
25 nd1.fixDOF('uy') # vertical support right end
26
27 # add loads
28 # .. load only the upper nodes
29 nd2.setLoad((0.0, -1.0), ('ux','uy'))
Line 5 instantiates one model space.
Lines 8-10 create the nodes, and lines 12-14 add them to the model space.
Lines 16-19 create the elements and simultaneously adds them to the model space. You only need to create variables for Node and Element objects, respectively, if you need to either add or retrieve information from that object later.
Lines 21-25 define the support conditions by providing the respective information directly to the supported nodes.
Lines 27-29 applies the reference load(s) as a nodal force at nd2.
The system equations are solved by a single call to the solver:
30 # analyze the model
31 model.solve()
You can obtain a debug-style report on the state of the system:
32 # write out report
33 model.report()
Resulting in an output like (may change as the code evolves).
System Analysis Report ======================= Nodes: --------------------- Node 0: {'ux': 0, 'uy': 1} x:[0. 0.], fix:['ux', 'uy'], P:[0. 0.], u:[0. 0.] Node 1: {'ux': 0, 'uy': 1} x:[72. 0.], fix:['uy', 'ux'], P:[0. 0.], u:[0. 0.] Node 2: {'ux': 0, 'uy': 1} x:[36. 36.], fix:[], P:[ 0. -1.], u:[ 0. -5.09116882] Elements: --------------------- Truss: node 0 to node 1: material properties: FiberMaterial(Material)({'E': 10.0, 'A': 1.0, 'nu': 0.0, 'fy': 1e+30}) strain:0.0 stress:{'xx': 0.0, 'yy': 0.0, 'zz': 0.0, 'xy': 0.0} internal force: 0.0 Pe: [ 0.0 0.0 ] Truss: node 0 to node 2: material properties: FiberMaterial(Material)({'E': 10.0, 'A': 1.0, 'nu': 0.0, 'fy': 1e+30}) strain:-0.06989658167930027 stress:{'xx': -0.6989658167930026, 'yy': 0.0, 'zz': 0.0, 'xy': 0.0} internal force: -0.6989658167930026 Pe: [ -0.530317996923979 -0.4553197065619366 ] Truss: node 1 to node 2: material properties: FiberMaterial(Material)({'E': 10.0, 'A': 1.0, 'nu': 0.0, 'fy': 1e+30}) strain:-0.06989658167930027 stress:{'xx': -0.6989658167930026, 'yy': 0.0, 'zz': 0.0, 'xy': 0.0} internal force: -0.6989658167930026 Pe: [ 0.530317996923979 -0.4553197065619366 ]
An easier way to look at the simulation results are plots. A deformed system plot is obtained using the model.plot() directive. If a filename is given, the plot will be saved to the harddrive using that file name.
34 # create plots
35 model.plot(factor=1., filename="truss03_deformed_a.png")
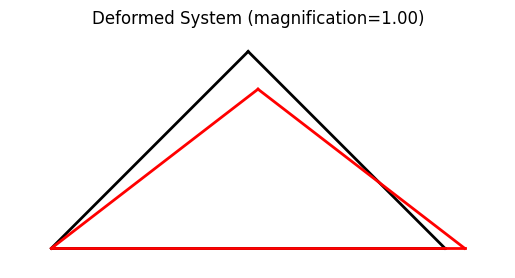
Showing file truss03_deformed_a.png
Analyzing a variation of this system
Studying a variation of a system is rather easy once a model has been generated. Let us modify the system by fixing the horizontal movement of node 1 in addition to the existing constraints (Line 37).
We also have to reset the displacements (Line 42) to reinitialize the analysis.
36 # fix horizontal motion of node 1
37 nd1.fixDOF('ux')
38
39 # add loads: same load -- nothing to do
40
41 # RE-analyze the model
42 model.resetDisp()
43 model.solve()
44
45 # skip the report
46 model.report()
47
48 # create plots
49 model.plot(factor=1., filename="truss03_deformed_b.png")
50
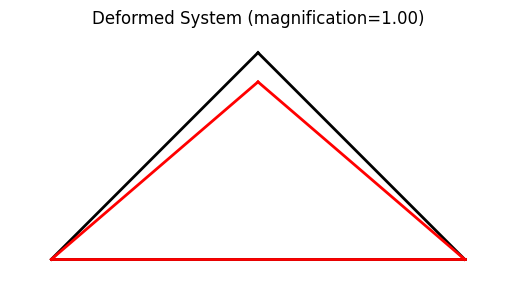
Showing file truss03_deformed_b.png. Note that neither of the bottom nodes moves after modifying the support.
Importing the example
You can import and run this example from the distribution as follows.
from femedu.examples.trusses.truss03 import *
# load the example
ex = ExampleTruss03()
More truss examples: Truss Examples